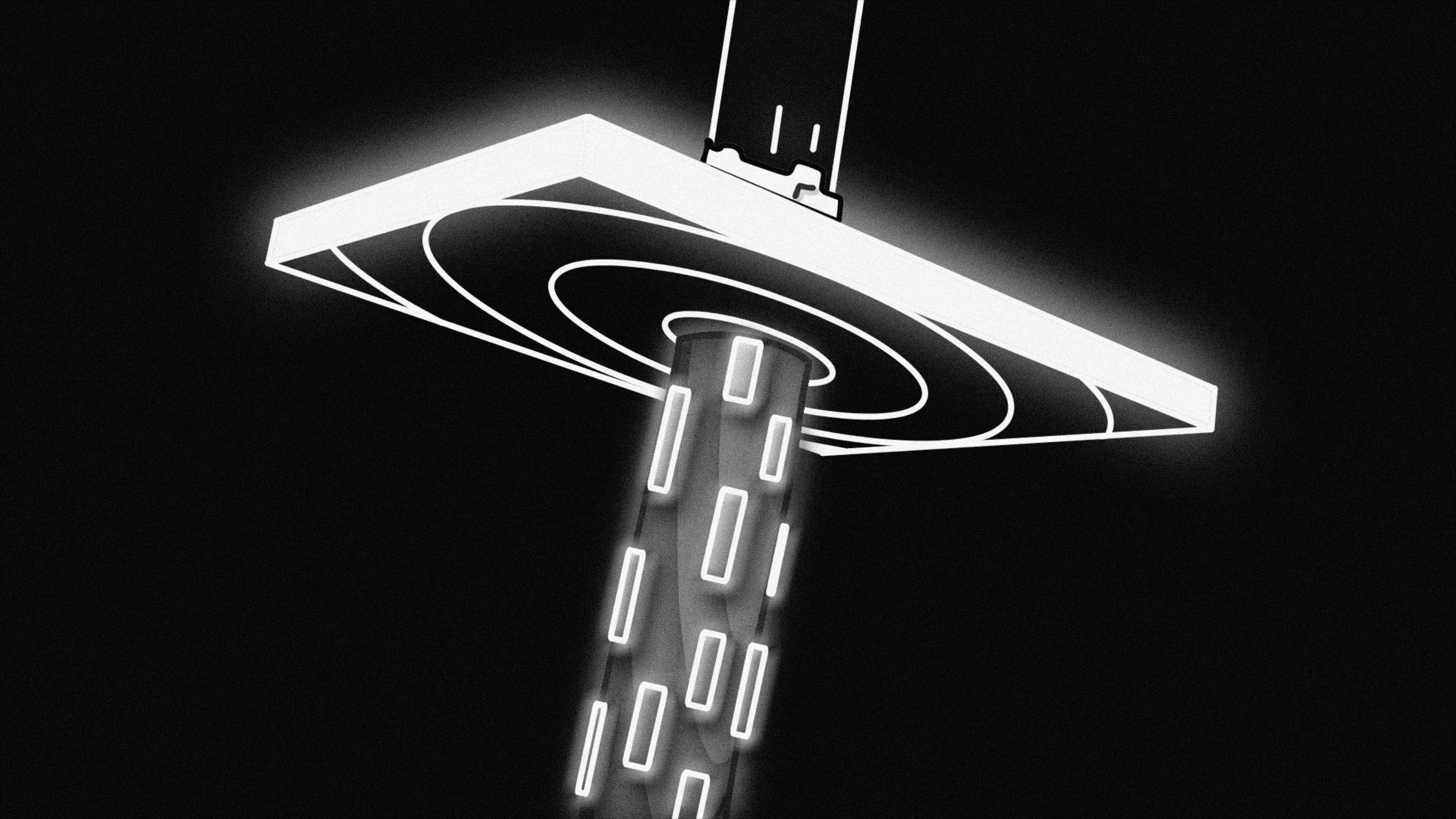
AI Starter Guide for Builders
As a software developer venturing into AI integration, you're likely wondering how to enhance your applications with AI capabilities without getting lost in the complexity. This guide will walk you through practical approaches to adding various AI features to your applications, with a focus on getting up and running quickly while making sustainable architectural decisions.
(Note: You can find references to the tools mentioned in the resources section.)
Generative AI Features
Quick Solution:
- Start with OpenAI's API or Anthropic's Claude API
- Use LangChain for orchestration
- Store conversations in MongoDB or PostgreSQL
Open Source Alternative:
- Use Ollama to run Mistral or Llama models locally
- Implement vLLM for efficient inference
- Use ChromaDB for vector storage
Implementation Steps:
- Set up a FastAPI backend
- Implement conversation history storage
- Add vector storage for context
- Deploy using Modal or Replicate for scalability
1from langchain.chat_models import ChatOpenAI
2from langchain.memory import MongoDBChatMessageHistory
3from langchain.chains import ConversationChain
4
5# Initialize chat model
6chat = ChatOpenAI()
7# Set up conversation memory
8memory = MongoDBChatMessageHistory(
9 connection_string="mongodb://localhost:27017/",
10 session_id="user-123"
11)
12# Create conversation chain
13conversation = ConversationChain(
14 llm=chat,
15 memory=memory,
16 verbose=True
17)
18
Quick Solution:
- DALL-E 3 API for highest quality
- Stable Diffusion for cost-effectiveness
- Store images in Amazon S3 or Azure Blob Storage
Open Source Alternative:
- Self-hosted Stable Diffusion using AUTOMATIC1111's web UI
- Operate through REST API
- Use Redis for caching
Implementation Steps:
- Set up image generation endpoint
- Implement prompt validation
- Add result caching
- Set up CDN for delivery
Quick Solution:
- OpenAI Whisper API for transcription
- ElevenLabs for text-to-speech
- Store audio files in cloud storage
Open Source Alternative:
- Self-hosted Whisper using whisper.cpp
- Mozilla TTS for speech synthesis
- Local file storage with CDN
Quick Solution:
- Replicate for hosted video models
- Genmo for quick integration
- Cloud storage for video files
Open Source Alternative:
- Self-hosted Stable Diffusion for frame generation
- FFMPEG for video compilation
- Implement caching layer
Natural Language Processing
Quick Solution:
- OpenAI API for complex tasks
- Cohere for specialized tasks
- MongoDB for document storage
Open Source Alternative:
- Sentence Transformers for embeddings
- spaCy for basic NLP
- PostgreSQL with pgvector
1from sentence_transformers import SentenceTransformer
2import spacy
3
4# Load models
5embedder = SentenceTransformer('all-MiniLM-L6-v2')
6nlp = spacy.load("en_core_web_sm")
7
8# Generate embeddings
9text = "Your text here"
10embedding = embedder.encode(text)
11
12# Basic NLP
13doc = nlp(text)
14entities = [(ent.text, ent.label_) for ent in doc.ents]
Computer Vision
Quick Solution:
- Azure Computer Vision
- Google Cloud Vision API
- Store images in cloud storage
Open Source Alternative:
- OpenCV for image processing
- YOLOv8 for object detection
- Local processing with GPU acceleration
Architecture Considerations
1User Request → API Gateway (Kong/FastAPI)
2 → Model Serving Layer (vLLM/TorchServe)
3 → Vector Store (ChromaDB/Pinecone)
4 → Storage Layer (PostgreSQL/MongoDB)
Key Components:
- API Layersome text
- FastAPI for quick development
- Kong for API gateway
- Redis for caching
- Model Servingsome text
- vLLM for LLM serving
- TorchServe for ML models
- BentoML for deployment
- Storagesome text
- PostgreSQL with pgvector for structured data
- MongoDB for document storage
- ChromaDB for vector storage
- Monitoringsome text
- Prometheus for metrics
- Grafana for visualization
- WhyLabs for ML monitoring
Cost Considerations
Hosted Solutions vs. Open Source
When to Use Hosted Solutions:
- Rapid prototyping
- Small to medium scale
- Limited ML expertise
- Time-to-market priority
When to Use Open Source:
- Large scale deployments
- Data privacy requirements
- Cost sensitivity
- Customization needs
Cost Optimization Strategies
- Tiered Processing:some text
- Use smaller models for simple tasks
- Reserve larger models for complex queries
- Implement caching aggressively
- Hybrid Approach:some text
- Run basic models locally
- Use cloud APIs for complex tasks
- Cache frequent requests
- Infrastructure:some text
- Use spot instances for batch processing
- Implement auto-scaling
- Optimize model serving
Feature | Hosted Solution | Open Source |
---|---|---|
Chatbot | $0.01-0.03/1K tokens | Server costs only |
Image Gen | $0.02-0.04/image | GPU server costs |
Audio | $0.006/minute | CPU server costs |
NLP | $0.01-0.02/1K tokens | Server costs only |
- Begin with one AI feature
- Use hosted solutions initially
- Implement proper monitoring
- Move to self-hosted as needed
- Optimize based on usage patterns
- Monitor costs and performance
- Implement robust error handling
- Add usage monitoring
- Set up cost alerts
- Regular model evaluation
When adding AI features to your application, start with hosted solutions for quick implementation and gradually move to open-source alternatives as your needs grow. Focus on proper architecture from the start, emphasizing scalability and cost management. Remember that the AI landscape is rapidly evolving, so design your system to be modular and adaptable to new technologies.